Preview and Customization
Before creating real Stripe subscription
products, you may want to design the /pricing
page first.
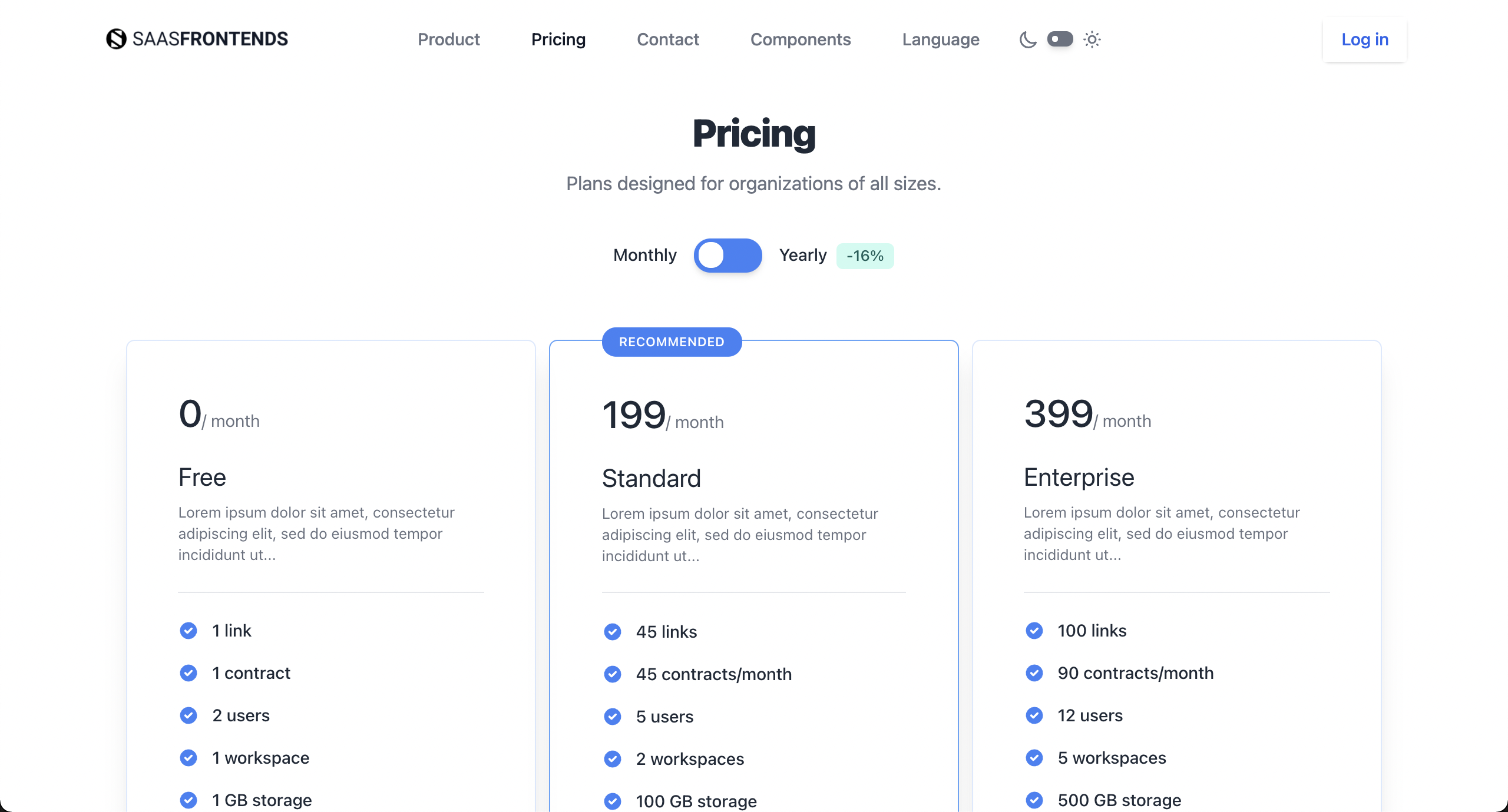
Configuration
You can customize them at application/pricing/plans.ts
.
plans.ts
import { SubscriptionProductDto } from "@/application/dtos/core/subscriptions/SubscriptionProductDto";
import { SubscriptionBillingPeriod } from "@/application/enums/core/subscriptions/SubscriptionBillingPeriod";
import { SubscriptionPriceType } from "@/application/enums/core/subscriptions/SubscriptionPriceType";
const currency = process.env.VUE_APP_CURRENCY?.toString() ?? "usd";
const plans: SubscriptionProductDto[] = [
{
serviceId: "",
id: undefined,
tier: 1,
title: "pricing.products.plan1.title",
description: "pricing.products.plan1.description",
contactUs: false,
prices: [
{
serviceId: "",
id: undefined,
type: SubscriptionPriceType.RECURRING,
billingPeriod: SubscriptionBillingPeriod.MONTHLY,
price: 0,
currency,
trialDays: 0,
active: true,
},
{
serviceId: "",
id: undefined,
type: SubscriptionPriceType.RECURRING,
billingPeriod: SubscriptionBillingPeriod.YEARLY,
price: 0,
currency,
trialDays: 0,
active: true,
},
],
features: [
{
id: undefined,
order: 1,
key: "pricing.features.oneLink",
value: "1",
included: true,
},
{
id: undefined,
order: 2,
key: "pricing.features.oneContract",
value: "1",
included: true,
},
{
id: undefined,
order: 3,
key: "pricing.features.maxUsers",
value: "2",
included: true,
},
{
id: undefined,
order: 4,
key: "pricing.features.oneWorkspace",
value: "1",
included: true,
},
{
id: undefined,
order: 5,
key: "pricing.features.maxStorage",
value: "1 GB",
included: true,
},
{
id: undefined,
order: 6,
key: "pricing.features.prioritySupport",
value: "",
included: false,
},
],
badge: "",
active: true,
maxUsers: 2,
maxWorkspaces: 1,
maxLinks: 1,
maxStorage: 1024,
monthlyContracts: 1,
},
...
];
export default plans;
Localization
Keep in mind that every hard-coded string is translated at runtime. For example for pricing.products.plan1.title
, you would need and entry at en-US.json
and es-MX.json
locale files.
en-US.json
...
"pricing": {
...
"products": {
"plan1": {
"title": "Free",
...
},
"plan2": {
"title": "Standard",
...
Frontend Environment
You need to set up the following environment variables:
- .env.development: ...APP_SUBSCRIPTION_PUBLIC_KEY
- .env.development: ...APP_SUBSCRIPTION_SECRET_KEY
- .env.production: ...APP_SUBSCRIPTION_PUBLIC_KEY
- .env.production: ...APP_SUBSCRIPTION_SECRET_KEY
.env.development
...
..._APP_SUBSCRIPTION_PUBLIC_KEY=pk_test_...
..._APP_SUBSCRIPTION_SECRET_KEY=sk_test_...
Backend Environment
And if you have the .NET backend, you also need to configure:
- .appsettings: "SubscriptionSettings"."PublicKey"
- .appsettings: "SubscriptionSettings"."SecretKey"
- .appsettings.Development: "SubscriptionSettings"."PublicKey"
- .appsettings.Development: "SubscriptionSettings"."SecretKey"
- .appsettings.Testing: "SubscriptionSettings"."PublicKey"
- .appsettings.Testing: "SubscriptionSettings"."SecretKey"
appSettings.json
...
"SubscriptionSettings": {
"PublicKey": "pk_test_...",
"SecretKey": "sk_test_..."
},